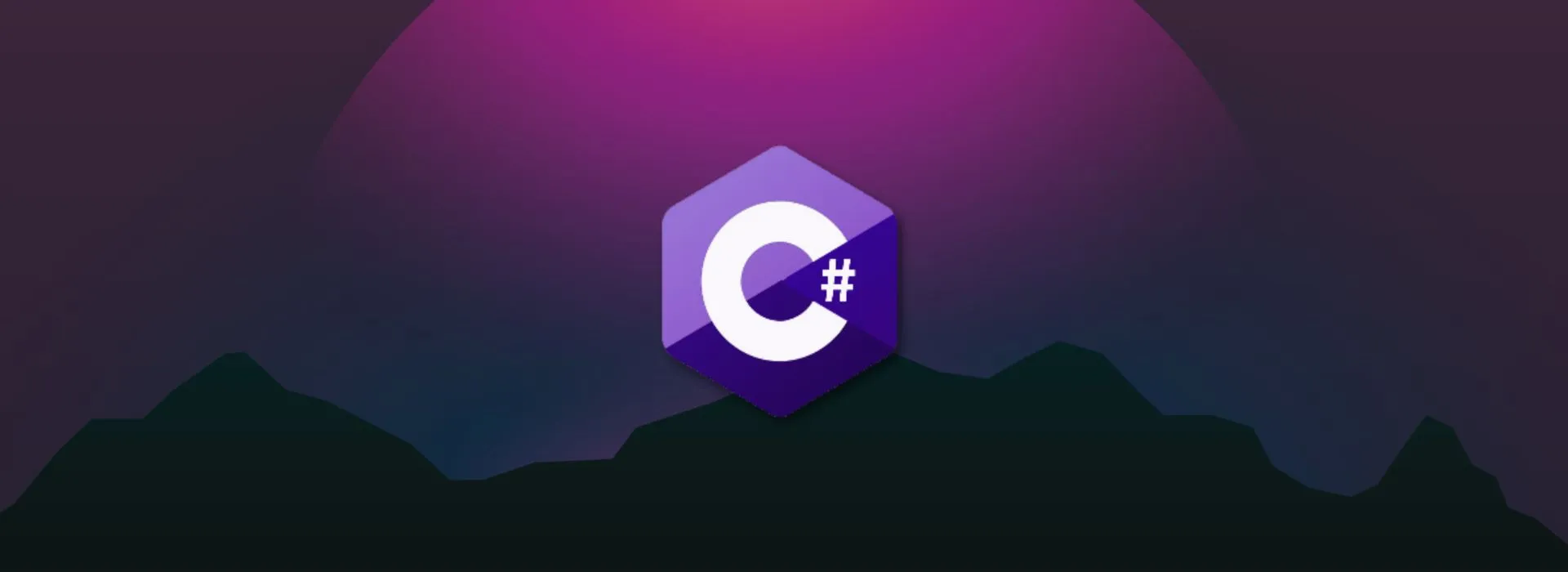
C# 8.0 ??= Null Coalescing Operators & Encapsulation
Hi, in today’s article, I will share my experiences using the ??= operator that came with C# 8.0.
Sometimes in C# we mean that if an object is empty then set to another object otherwise we want to continue using that object.
Now let’s make an example.
This operator briefly functions as assign the right object if the left object is empty, otherwise return the left one.
for example we have a property named Name.
public string Name {get;set;}
When we try to access this property while it is empty, we will encounter vs ArgumentNullException when we try to convert it. We also have a check like (Name != Null) so that the program does not give an error. For this and similar cases, we need to say if it is null, do this, otherwise, do this.
For example, you will pull the user’s name with the id found in the session (not real usage).
private string name;
public string Name {
get {
if (name == null) {
name = datacontext.users.FirstOrDefault(u => u.UserId == sessionID).name;
}
return name;
}
}
Thanks to the above process, we pulled the user name from the database and assigned it to the private variable, so we also prevented going to the database when trying to access the name again.
So how do we shorten this code, so short if was introduced?? operator came into our lives with C# 7.
private string name;
public string Name {
get {
return name ?? (name = datacontext.users.FirstOrDefault(u => u.UserId == sessionID).name);
}
}
We don’t need if blocks anymore in this case. The point you need to pay attention to here is that you cannot assign the right result directly to the left. If the left is blank, it executes the code on the right. For this reason, there is an assignment in the form of name = in the right field.
Now let’s come to the use of ??= ;
private string name;
public string Name {
get {
return name ??= datacontext.users.FirstOrDefault(u => u.UserId == sessionID).name;
}
}
Here, we have said that if the object is empty, fill it with this.
Let’s look at how we use it in a real project.
In an online exam system I developed, I prepared the DataContext with linq2db, added it to the scope via startup, and opened a separate class called Participant. This class also requests datacontext in the constructor phase.
Here, I request the user’s answers on the methods more than once and look at the situations such as what questions the user answered with commands such as any, count etc.
If I don’t encapsulate, every time I call Answers, I will have pulled data from the database again. I had to do a simple encapsulation as I would reach it more than once in the same page. I was able to do this very simply with the ??= operator.
private List <UserAnswer> _answers;
public List <UserAnswer> Answers => _answers ??= _connection.UserAnswers.Where(w => w.UserID == ParticipantID).ToList();
I’m thinking of preparing and posting a video about linq2db soon. I can say that I have never seen such a simple, effective and fast ORM on .Net Core, if anyone is already curious, they can have a look by clicking here .
If you want to learn more about Null Coalescing Operators, you can refer to Microsoft Docs .
Do not forget to comment and express your thoughts.
Have a great day 👋